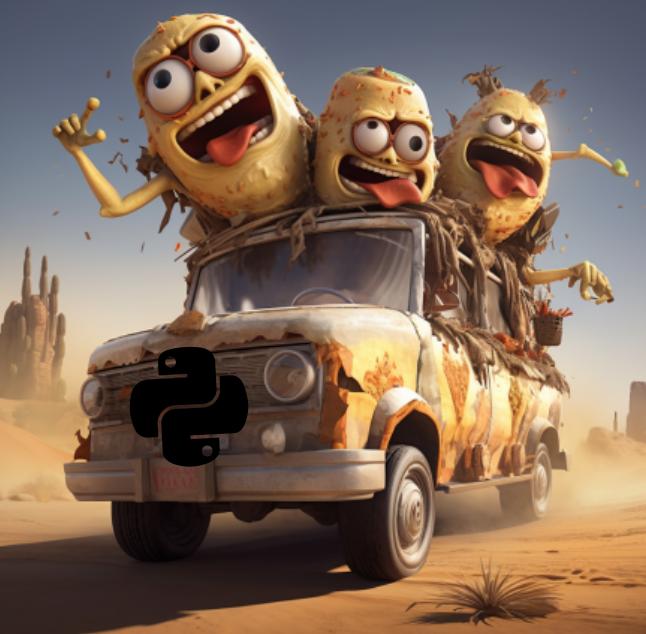
U Pythonu, koristimo tipove podataka kao način za određivanje očekivanih tipova varijabli, parametara funkcije i povratnih vrijednosti. Tako da naprimjer, imamo funkciju koja zbraja dva broja.Nama je bitno da kao argument primamo samo numeričke tipove podataka. Tako da je dovoljno da samo u argumentu postavimo da je dozvoljeni tip argumenta integer ili float. Što znači da nema potrebe da provjeravamo tip podataka unutar funkcije sa “if type(num) == int:”. Isto tako, možemo odrediti povratni tip funkcije.
Da biste koristili hintiranje tipa u Pythonu, trebate označiti varijable, parametre funkcije i povratne vrijednosti s odgovarajućom oznakom tipa.
Tipovi podataka
Primitivni tipovi
int | cijeli broj |
float | broj s pomičnim zarezom |
bool | Boolean (true/false) |
str | string |
bytes | bajtovi |
Collections / Iterables tipovi
list | List |
tuple | Tuple |
set | Set |
frozenset | Immutable set |
dict | Dictionary |
Iterable | Generic iterable type hint |
Sequence | Generic iterable type hint |
Mapping | Generic mapping type hint |
Drugi Built-in tipovi
None | NoneType (predstavlja odsutnost vrijednosti) |
Any | Tipska oznaka koji pokazuje da je bilo koji tip dopušten |
Union | Tipska oznaka koji ukazuje na mogučnost korištenja više tipova |
Optional | Tipska oznaka koja označava specificiranu vrstu ili None |
Callable | Tipska oznaka za objekt koji se može pozvati (funkcije, metode itd.) |
Generator | Tipska oznaka za funkciju/expression generatora |
Type | Tipska oznaka za vrstu (npr. int, str, MyClass) |
AnyStr | Tipska oznaka za objekt nalik nizu (bajtovi ili string) |
TypeVar | Tipska oznaka za stvaranje generičkih tipova i varijabli tipa |
Vrste specifične za module
datetime | vrste povezane s datumom i vremenom (npr. datetime.datetime, datetime.date) |
decimal | decimalni brojevi (npr. decimal.Decimal) |
typing | Modul za definiranje i rad s tipovima (npr. typing.List, typing.Dict, typing.Union) |
Primjeri
Rad sa varijablama:
age: int = 25 name: str = "John" is_valid: bool = True
Rad sa funkcijama:
def greet(name: str) -> None: print("Hello, " + name) def add(x: int, y: int) -> int: return x + y
Collections/Iterables:
from typing import List, Tuple, Set, FrozenSet, Dict, Iterable, Sequence, Mapping # List of integers numbers: List[int] = [1, 2, 3, 4, 5] # Tuple of strings and integers person: Tuple[str, int] = ("Alice", 25) # Set of floating-point numbers scores: Set[float] = {98.5, 87.2, 92.0} # Immutable set of strings fruits: FrozenSet[str] = frozenset(["apple", "banana", "orange"]) # Dictionary with string keys and integer values ages: Dict[str, int] = {"Alice": 25, "Bob": 30, "Charlie": 35} # Generic iterable data: Iterable[int] = [1, 2, 3, 4, 5] # Generic sequence sequence: Sequence[str] = ("a", "b", "c") # Generic mapping mapping: Mapping[str, int] = {"a": 1, "b": 2, "c": 3}
Built-in:
from typing import Optional, Union, Any, Callable, Generator, Type, AnyStr, TypeVar # None: NoneType (represents absence of value) result: Optional[int] = None # Any: Type hint indicating any type is allowed value: Any = "Hello" # Union: Type hint indicating multiple possible types score: Union[int, float] = 98.5 # Optional: Type hint indicating either a specified type or None name: Optional[str] = "Alice" # Callable: Type hint for a callable object (functions, methods, etc.) function: Callable[[int, str], bool] = some_function # Generator: Type hint for a generator function or generator expression generator: Generator[int, None, str] = some_generator() # Type: Type hint for a type (e.g., int, str, MyClass) class_type: Type[MyClass] = MyClass # AnyStr: Type hint for a string-like object (bytes or str) text: AnyStr = "Hello" # TypeVar: Type hint for creating generic types and type variables T = TypeVar('T') # Type variable representing a generic type value: T = some_value
Moduli:
from datetime import datetime, date from decimal import Decimal from typing import List, Dict, Union # datetime: Date and time related types current_time: datetime = datetime.now() current_date: date = date.today() # decimal: Decimal numbers price: Decimal = Decimal('19.99') tax_rate: Decimal = Decimal('0.08') # typing: Module for defining and working with types user_ids: List[int] = [1, 2, 3, 4, 5] person: Dict[str, Union[str, int]] = {"name": "Alice", "age": 25}